We can use screen, tmux, nohup command to run process in background.
But, what we can do if we already run the process and we want to send it to background.
We can use kill signal to achieve this.
kill -SIGSTOP PID
kill -SIGCONT PID
SIGSTOP = pause the process
SIGCONT = continue the process
Here is example.
sleep-loop.sh
while(true)
do
echo "running loop...$(date +%s)"
sleep 5
done
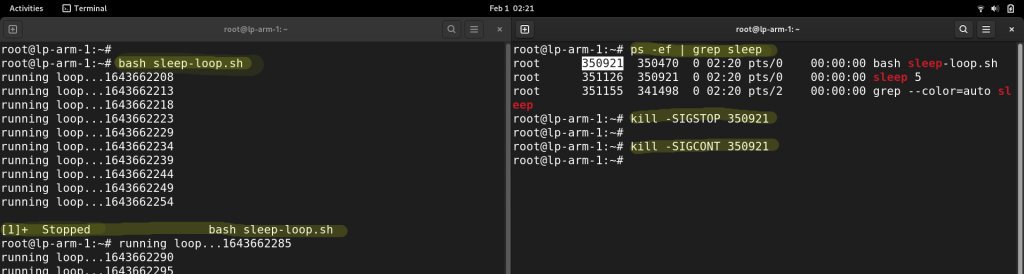
You can close the 1st terminal after starting in running the SIGCONT in another terminal AND you can also close the 2nd second terminal as well as it’s running in backgroud.(kind of nohup)
Other method using jobs command
- Press CTRL + Z to Pause the current process
- bg = to send job to background
disown %1
disow will remove form job queue and run in background so that we can close the terminal
More – https://stackoverflow.com/questions/625409/how-do-i-put-an-already-running-process-under-nohup