pipeline {
agent {label 'master'}
parameters {
string(name: 'string1', defaultValue: 's1', description: 's1')
choice(name: 'CHOICES', choices: ['one', 'two', 'three'], description: 'chose')
password(name: 'password', defaultValue: 'SECRET', description: 'password')
}
stages{
stage('one'){
steps{
sh "echo abc > abc.txt"
stash includes: 'abc.txt', name: 'abc'
sh "rm -rf abc.txt"
}
}
stage('two'){
steps{
unstash 'abc'
sh "cat abc.txt"
}
}
}
}
Category: Uncategorized
Jenkins stash and unstash
pipeline {
agent {label 'master'}
stages{
stage('one'){
steps{
sh "echo abc > abc.txt"
stash includes: 'abc.txt', name: 'abc'
sh "rm -rf abc.txt"
}
}
stage('two'){
steps{
unstash 'abc'
sh "cat abc.txt"
}
}
}
}
Open port using NC command in linux
Open port using NC:
nc 8888
Listen port:
nc localhost 8888
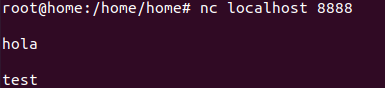
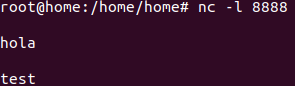
Send packet using bash:
echo -n "hello" >/dev/tcp/localhost/8888
Mount cifs/samba share inside container
#Dockerfile
FROM ubuntu
RUN apt update -y
RUN apt install cifs-utils -y
docker build -t cifs .
docker run -it --cap-add SYS_ADMIN --cap-add DAC_READ_SEARCH -cap-add NET_BIND_SERVICE cifs bash
#centos with privileged (working)
docker run --privileged -it --cap-add SYS_ADMIN --cap-add DAC_READ_SEARCH --cap-add NET_BIND_SERVICE centos bash
mount -t cifs -o username=a,password=a //192.168.0.228/public /mnt
mount -t cifs -o username=a,password=a //192.168.0.228/public /mnt
mount -t cifs -o username=a,password=a,ro,domain=WORKGROUP //192.168.0.228/public /a -v
Get aws ec2 instance id from shell/bash
cat /sys/devices/virtual/dmi/id/board_asset_tag
#short hostname
hname=$(cat /sys/devices/virtual/dmi/id/board_asset_tag | awk '{print substr($0,5,15)}')
hostnamectl set-hostname $hname
Mysql allow from all – Test
CREATE USER 'root'@'%' IDENTIFIED BY 'Test#123';
GRANT ALL PRIVILEGES ON *.* TO 'root'@'%' WITH GRANT OPTION;
Jenkins questions list
- install jenkins using docker-compose
- default port for jenkins 8080
- Explain the your CICD pipeline
- What is DSL?
- How do you manage credentials in jenkins?
- explain the basic structure of Jenkinsfile?
- how jobs are managed for different branch/ multibranch?
- What issues you faced in jenkins? = plugin high disk IO
- build trigger?
- how to configure webhook?
- poll SCM?
- light checkout in jekins?
- groovy sandbox?
- add worker node in jenkins? types of method?
Disk IO read/write monitor
yum install sysstat -y
iostat -t
iostat -xtc
iostat -d
#More command
iostat -dx /dev/sda 5
sar
vmstat
badblock
Sonatype Nexus3 – Docker compose
version: '3'
services:
jenkins:
image: sonatype/nexus3:3.29.0
user: root:root
restart: always
container_name: nexus
environment:
TZ: "Asia/Kolkata"
volumes:
- /opt/nexus-data:/nexus-data
ports:
- 8081:8081
Get admin passwords:
docker exec -it nexus bash
find / -iname admin.*
#OR
docker exec -it nexus cat /nexus-data/admin.password
More : https://hub.docker.com/r/sonatype/nexus3#user-content-persistent-data
Nomad – Container orchestration example for Dev environment
#Install nomad
-Download the stable release form https://www.nomadproject.io/downloads
wget https://releases.hashicorp.com/nomad/1.0.1/nomad_1.0.1_linux_amd64.zip
unzip nomad_1.0.1_linux_amd64.zip
mv nomad /usr/local/bin/
#start nomad in dev mode
nomad agent -dev
nomad node status
#Install docker https://docs.docker.com/engine/install/centos/
yum-config-manager \
--add-repo \
https://download.docker.com/linux/centos/docker-ce.repo
yum install docker-ce docker-ce-cli containerd.io
systemctl start docker
systemctl enable docker
#Create simple nginx job file (nginx.nomad)
job "nginx" {
datacenters = ["dc1"]
type = "service"
update {
max_parallel = 1
min_healthy_time = "10s"
healthy_deadline = "3m"
progress_deadline = "10m"
auto_revert = false
canary = 0
}
migrate {
max_parallel = 1
health_check = "checks"
min_healthy_time = "10s"
healthy_deadline = "5m"
}
group "cache" {
count = 1
network {
port "nginx-port" {
to = 80
}
}
service {
name = "nginx-port"
tags = ["nginx", "web"]
port = "nginx-port"
}
restart {
attempts = 2
interval = "30m"
delay = "15s"
mode = "fail"
}
ephemeral_disk {
size = 300
}
task "nginx" {
driver = "docker"
config {
image = "nginx"
ports = ["nginx-port"]
}
resources {
cpu = 500
memory = 256
}
}
}
}
#Nomand commands for Run,Stop, job status and logs
nomad job status
nomad job run nginx.nomad
nomad job stop nginx
nomad job status nginx
nomad alloc status <Allocations ID>
nomad alloc logs <Allocations ID>
#Access webUI at http://127.0.0.1:4646